Bash Scripts
audio_download
This script utilizes yt-dlp to fetch only the audio from a youtube video or playlist. I don't listen to a ton of music, so this is an easy way for me to grab an album off YouTube. I don't have to worry about accounts or managing a library. I just get cold, hard files. It's also great for meme songs that don't appear elsewhere.
yt-dlp --audio-quality best -x $1
discord_notify
This script utilizes curl to ping a Discord webhook. In Discord, right click a server that you own and go to "Integrations". Here you can set up a webhook. It'll have a bot name and a url. Whatever channel it is set to is where your message will land.
curl -i -H "Accept: application/json" -H "Content-Type:application/json" -X POST --data "{\"content\":\"$*\"}" https://theurlofyourwebhook
I have Discord on my phone and use this as a lazy way to send notifications to myself. It's very easy to run a long command and append this.
compile && discord-notify "Compilation is complete"
If I forget to append it, I can use ctrl+z to put the command into the background and then run 'fg && discord-notify "Compilation is complete"'. It's much easier and more lightweight than a bot. This is also what pings Discord inbetween Dominions 5 turns.
get_yoga
I get analysis paralysis super bad when it comes to finding instructional videos. I also need to stretch or my spine will cripple for months. I will get bored if I watch the same video twice. My solution : a script that grabs a random yoga video from a popular YouTuber. No questions of whether it's suitable, easy, or hard. I just get a video and then I can do that.
number=$((1 + $RANDOM % 600))
yt-dlp -o /home/fk/Videos/yoga.webm --playlist-items $number https://www.youtube.com/@yogawithadriene/videos
vlc /home/fk/Videos/yoga.webm
As you can see, there are 600+ videos on Adriene's channel. We generate a number between 1 and 600. We get that video. We play that video immediately. The end result is that "get_yoga" gives me yoga to do. Be kind to your spine.
projsum & summarize
projsum is the command I use to invoke a script called "summarize" on my Projects folder. summarize uses glow to make the output pretty, but it's not necessary at all. This is all about grep and the beauty of formatted text files.
echo '' > summary
if [ "$1" == "" ]; then
list=(*/*notes)
fi
for i in "${list[@]}"; do
echo $(head -q -n 1 $i) >> summary
grep -A5 ToDo $i | grep -v '#' >> summary
echo -e '\n' file://$(realpath $i) >> summary
done
glow summary
We are in the folder Projects. Inside this folder is a folder for each of my projects. Inside each of those folders is a "notes" file. Inside the ~/Projects/ray_snake/notes file is a section like so :
#Ray Snake
Snake made in C using raylib.
#ToDo
1. Display a sprite
1. Display the game board
1. Food
1. Randomize Placement
1. Snake
1. Input
1. Collision
1. Snake grows
1. Snake hinges
1. Lose
1. Music
1. Gobble / Die sound effects
1. Controller support
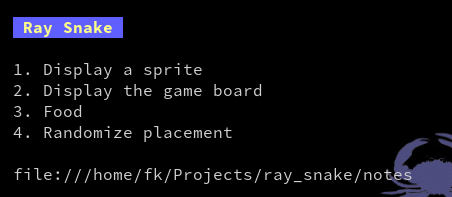
The output summarizes only the top few items, to avoid brain overload. I can put as many unrelated notes in the file as I want. It only looks for the the first line and #ToDo sections. It also provides a clickable link (opens in vim) to the notes file of that project. This script will output one long list of every project in the Projects folder. It's an easy at-a-glance way to see where I left everything. Updating notes files is not a tedious task because of the clickable link.
internet_watch
Our internet went out a few times and I found it annoying to keep checking my PC to see if it was back on. I just wanted some kind of ping, when it was ready.
target="website.crabsoft.download"
state="offline"
clear
while true;
do
if [[ $state == "offline" ]]; then
if [[ $( ping -c 1 $target | grep "100%" ) != "" ]];then ##if we're offline and ping fails, log disconnect
time=$(date) ##this will show the time of last reconnect attempt
echo -e $time : '\033[0;31;48m'Disconnected'\033[1;37;0m';
else ##if ping succeeds, set us to online and send the notice
state="online"
echo -e $time : '\033[0;36;48m'Reconnected'\033[1;37;0m'
sleep 10;
discord-notify "Internet Restored";
fi
elif [[ $state == "online" ]]; then
if [[ $( ping -c 1 $target | grep "100%" ) != "" ]];then ##if we're already online and the ping fails, log disconnect and notify
state="offline" ## if ping succeeds, do nothing. this will only show the success reconnect time
echo -e $time : '\033[0;31;48m'Disconnected'\033[1;37;0m'
notify-send "Internet Disconnected";
fi;
fi
sleep 10;
done
I'm sure there's an easier way but this is reality. We just spam pings until we reach our destination and then discord-notify, which also goes to my phone. It prints out timestamps of the last time it pinged in nice red letters, so that I don't worry about if it's still working. I put this up on my wife's TV screen, so she could stop asking me lmao.
quittr
Quittr has had many iterations over the years. The concept is simple : a command that either logs or retrieves the reason you stopped doing something. I found myself constantly returning to games that I couldn't remember why I quit. I was swiftly reminded. Quittr is an attempt to skip that painful middle phase. If I have logged something in quittr, and that specific issue has not been resolved since I last visited, I don't bother.
quitfile="/home/fk/.bin/quits.txt"
if [ $# -eq 0 ]; then
cat $quitfile
elif [ $# -eq 1 ]; then
list=$(grep "${1}: " $quitfile)
list=${list#$1:}
list=$(echo $list | sed -e 's/\,/\\n/g')
echo -e ${list}
else
topic="${1}: "
if [ -z "$(grep $topic $quitfile)" ]; then
old=$topic
else
old=$(grep $topic $quitfile)
old=$old,
fi
sed -i "/$topic/d" $quitfile
new="${old}${2}"
echo $new >> $quitfile
fi
If no arguments, list all the quits. If one argument, list the quits for that specific topic. If more than one argument, add it to the list for that topic.
Assorted Aliases
I find scripts in the ~/.bin folder to be more manageable than proper aliases. I have a random assortment of scripts that just call normal commands with specific parameters. No shame. Here's "net_map".
nmap -sP 192.168.1.0/24
My rule is that if I'm likely to forget how to do something or that a command exists, I put it in a script as documentation. I literally have one called "json_edit" that is just :
echo "the tool is called vd"
It's your system. Make it comfortable for you.